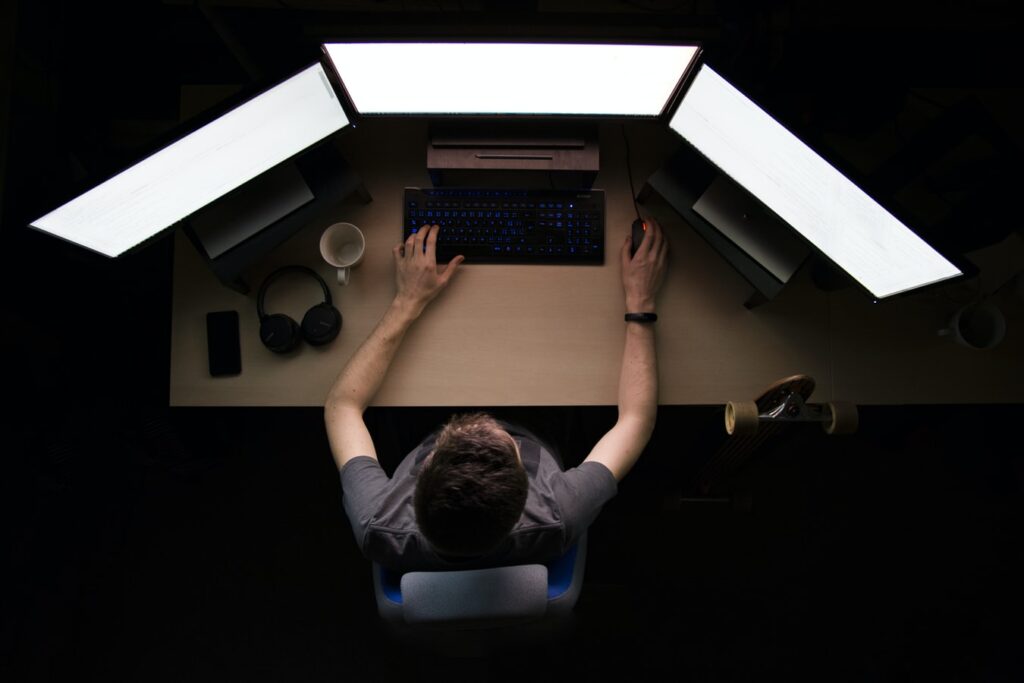
Adding unit tests to Microsoft C# projects can be a bumpy path to first timers, here are some guidelines to smooth the ride.
Create a new project, or open the existing project solution in Visual Studio. In the solution explorer, right-click on the solution, select Add then New Project. In the new window, filter the project type on category Test.
Select the correct project type depending on the type of project under test, either for .NET Core or.NET Framework applications
Select an appropriate name for the project (the recommendation is the same name as the project under test with _Test
appended).
Use the Nuget package manager to install the MSTest dependencies. Go to Tools
, NugetPackage Package Manager
, Manage Nuget Packages
for Solution. Search for MSTest
and then install MSTest.TestAdapter
and MSTest.TestFramework
.
The default test class should be already open in the IDE.
using System; using Microsoft.VisualStudio.TestTools.UnitTesting; namespace MyProject_Test { [TestClass] public class UnitTest1 { [TestMethod] public void TestMethod1() { } } }
Rename the TestClass to something more descriptive. Right click on UnitTest1
and select Rename to change the name of the test class. Modify the test method TestMethod1
in a similar fashion.
Add a reference to the new test project, for the project under test. Expand the test project and find the References
section. Right click and select Add Reference
. Under Projects
, Solution
there should already be the project under test. Check the enable box and click OK. Add a simple test to the first empty test method. Example:
using System; using Microsoft.VisualStudio.TestTools.UnitTesting; using MyProject; namespace MyProject_Test { [TestClass] public class IsNotNull_Test { [TestMethod] public void CreateObject() { MyObject myObj = new MyObject(); Assert.IsNotNull(myObject); } } }
As the targets under test fall in a different namespace, their access must be set to public. Declare any class under test as public.
Ensure the Platform Target for the test project is the same as that for the project under test, e.g. x86
, x64
or Any CPU
Ensure the target level of the platform is also the set the same, e.g. .NET Framework 4.6.1
.
Open the Test Explorer view and run all tests. The view should show the test passing.
Failing tests can be identified by the red cross next to the test method in the test explorer. Clicking on the failing method name will identify the line of the test failure.
To add console output to the tests, use the Console.WriteLine() method. The output does not show int he standard console output however. To see the output, first select the test method in the test explorer, then select the output link to show.
Tests can be single-stepped much like usual C# code. Adding and removing breakpoints happens in the same way by double clicking. Right click on the test project, then select debug_tests
.
TEST COVERAGE
Visual Studio Community Edition does not provide test coverage information. Thirdparty tools may be used to gather metrics on the code coverage.
The open source program OpenCover
may be used to generate test coverage metrics of a C#.NET application.
First, use the NuGet package manager within Visual Studio to install the packages OpenCover
and ReportGenerator
. OpenCover
provides only an .xml output file that must be then parsed with ReportGenerator
in order to nicely present the coverage results. Both applications are command line only, currently there is no IDE integration.
Build and run the tests in the normal way using the above as a guide. Enter the test solution folder and run OpenCover
:
C:\Users\%username%.nuget\packages\opencover\4.7.922\tools\OpenCover.Console.exe
Where:
The version of OpencCover
is correctly specified.
The -target
option points to the test harness rather than the compiled test program, here vstest
is used and is added to the system path for convenience. Usually it can be found here depending on Visual Studio version: C:\Program Files (x86)\Microsoft VisualStudio\2019\Community\Common7\IDE\CommonExtensions\Microsoft\TestWindow
-targetargs
specifies the built test application dll. There must also be a corresponding .pdb file in the same folder for OpenCover
to obtain debug information.
Hopefully running this command generates an output similar to the following, and the output file CoverageReport.xml
is created:
Microsoft (R) Test Execution Command Line Tool Version 16.8.0 Copyright (c) Microsoft Corporation. All rights reserved. Starting test execution, please wait… A total of 1 test files matched the specified pattern. Passed IsNotNull_Test [1 ms] Test Run Successful. Total tests: 1 Passed: 1 Total time: 1 ms Committing… Visited Classes 1 of 1 (100.00) Visited Methods 1 of 1 (100.00) Visited Points 1 of 1 (100.0) Visited Branches 1 of 1 (100.00)
The report may then be converted to a user friendly html version with ReportGenerator:
C:\Users\%username%.nuget\packages\reportgenerator\4.8.4\tools\net47\ReportGenerator\